HTML5 Game Physics Acceleration And Elastic Collisions
This tutorial is the second in a series about HTML5 game physics. Game character movement becomes more realistic when acceleration is used. Acceleration is the rate at which the velocity of a game character changes with time.
The second tutorial will move the game character vertically downwards according to gravity. Air or fluid resistance will be provided by a set drag coefficient. The bottom will act as a non-moving object for collision with the moving game character.
This series is intermediate and assumes basic knowledge of physics and 2D graphics. The drag coefficient is based on the size and speed of the character. Elastic collisions conserves momentum and kinetic energy which is possessed due to motion.
This tutorial uses the HTML5 canvas tag and JavaScript.
- Tools are required:
- Text editor.
- Folder for web server.
- Browser to view output.
HTML5 Elastic Collisions File
<!-- HTML5_Game_Physics_Acceleration_And_Elastic_Collisions.html Copyright 2013 edward <http://Ojambo.com> This program is free software; you can redistribute it and/or modify it under the terms of the GNU General Public License as published by the Free Software Foundation; either version 2 of the License, or (at your option) any later version. This program is distributed in the hope that it will be useful, but WITHOUT ANY WARRANTY; without even the implied warranty of MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU General Public License for more details. You should have received a copy of the GNU General Public License along with this program; if not, write to the Free Software Foundation, Inc., 51 Franklin Street, Fifth Floor, Boston, MA 02110-1301, USA. --> <!DOCTYPE html> <html xml:lang="en" lang="en"> <head> <title>Ojambo.com HTML5 Game Physics Acceleration & Elastic Collisions</title> <meta charset="utf-8" /> <style type="text/css"> canvas#myCanvas{ width: 80%; height: 80%; background: green; } </style> </head> <body> <canvas id="myCanvas"></canvas> <script type="text/JavaScript"> //Position, Size & Velocity var rect = { x: 0, y: 0, w: 20, h: 20, vx: 0, vy: 0 } // Find Canvas Element var myCanvas = document.getElementById("myCanvas"); // Call getContext() method for 2D drawing var ctx = myCanvas.getContext("2d"); //Update Every 20 milliseconds setInterval(function() { // Draw Function draw(); }, 20); // Draw Function function draw(){ //Clear Display ctx.save(); ctx.clearRect(0,0, myCanvas.width, myCanvas.height); ctx.restore(); //Apply velocity to position (vx -> x) rect.x += rect.vx; rect.y += rect.vy; // Apply drag/friction to velocity rect.vx *= 0.99; rect.vy *= 0.99; // Apply gravity to velocity rect.vy += 0.25; // Collision if (rect.y + rect.h > myCanvas.height) { rect.y = myCanvas.height - rect.h; rect.vy = -Math.abs(rect.vy); } // Draw on the canvas ctx.save(); //Fill style red colour ctx.fillStyle="#FF0000"; //Rectangle ctx.fillRect(rect.x, rect.y, rect.w, rect.h); ctx.restore(); } </script> </body> </html>
The game character will be pulled downwards by gravity. The acceleration will be hampered by the drag coefficient. The bottom of the canvas is reached when the game character position is exactly above the bottom.
The bottom of the canvas acts as a non-moving object for collisions. The game character will bounce off the bottom of the canvas. The canvas height minus the game character height is the bottom of the canvas.
How to Use:
- Open Browser
- Observe the canvas.
- Observe the character moving down the canvas.
- Observe the impact of the collision.
Demonstration:
Ojambo.com HTML5 Game Physics Elastic Collision Tutorial
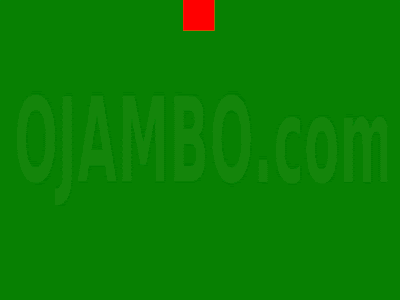
Conclusion:
Game characters accelerate downwards due to gravity. The drag coefficient provides air resistance for characters based on their size and velocity.
Elastic collisions cause characters to change their acceleration. The exact point of collision impact can be determined by the character’s position and dimensions.
- Recommendations:
- Determine which objects moves after a collision.
- Give large objects different drag coefficients.
- Use a character’s dimensions to determine collision aftermath.