HTML5 Game Physics Bouncing Elastic Collisions
This tutorial is the third in a series about HTML5 game physics. Game character movement becomes more realistic when multiple collisions are utilized. At each collision, a character will need to reverse or lose some momentum.
The third tutorial will move the game character in different directions after each collision. The bounce effect will be achieved by using a constant acceleration. The canvas sides will act as a non-moving object for collision with the moving game character.
This series is intermediate and assumes basic knowledge of physics and 2D graphics. The acceleration will hold steady but the velocity will be the exact opposite after each collision. Elastic collisions conserves momentum and kinetic energy which is possessed due to motion.
This tutorial uses the HTML5 canvas tag and JavaScript.
- Tools are required:
- Text editor.
- Folder for web server.
- Browser to view output.
HTML5 Moving Elastic Collisions File
<!-- HTML5_Game_Physics_Moving_Elastic_Collisions.html Copyright 2013 edward <http://Ojambo.com> This program is free software; you can redistribute it and/or modify it under the terms of the GNU General Public License as published by the Free Software Foundation; either version 2 of the License, or (at your option) any later version. This program is distributed in the hope that it will be useful, but WITHOUT ANY WARRANTY; without even the implied warranty of MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU General Public License for more details. You should have received a copy of the GNU General Public License along with this program; if not, write to the Free Software Foundation, Inc., 51 Franklin Street, Fifth Floor, Boston, MA 02110-1301, USA. --> <!DOCTYPE html> <html xml:lang="en" lang="en"> <head> <title>Ojambo.com HTML5 Game Physics Moving Elastic Collisions</title> <meta charset="utf-8" /> <style type="text/css"> canvas#myCanvas{ width: 80%; height: 80%; background: green; } </style> </head> <body> <canvas id="myCanvas"></canvas> <script type="text/JavaScript"> // Position, Size & Velocity var rect = { x: 0, y: 0, w: 20, h: 20, vx: 2, vy: 3 }; // Find Canvas Element var myCanvas = document.getElementById("myCanvas"); // Call getContext() method for 2D drawing var ctx = myCanvas.getContext("2d"); // Update Every 20 milliseconds setInterval(function() { // Draw Function draw(); }, 20); // Draw Function function draw(){ //Clear Display ctx.save(); ctx.clearRect(0,0, myCanvas.width, myCanvas.height); ctx.restore(); // Apply velocity to position (vx -> x) rect.x += rect.vx; rect.y += rect.vy; // Collision with canvas horizontally if ( rect.x > myCanvas.width - rect.w ) { rect.x = myCanvas.width - rect.w; rect.vx = -Math.abs(rect.vx); } else if ( rect.x < 0 ) { rect.x = 0; rect.vx = Math.abs(rect.vx); } // Collision with canvas vertically if ( rect.y > myCanvas.height - rect.h ) { rect.y = myCanvas.height - rect.h; rect.vy = -Math.abs(rect.vy); } else if ( rect.y < 0 ) { rect.y = 0; rect.vy = Math.abs(rect.vy); } // Draw on the canvas ctx.save(); //Fill style red colour ctx.fillStyle="#FF0000"; //Rectangle ctx.fillRect(rect.x, rect.y, rect.w, rect.h); ctx.restore(); } </script> </body> </html>
The game character will bounce of the top, bottom, left and right sides of the canvas. The velocity will be change by an equal amount every time. Movement from left to right or right to left will be at a constant acceleration.
Velocity will reverse when the game character bounces off a side of the canvas. The first if statement deals with the left to right and right to left collision. The second if statement deals with the top to bottom and bottom to top collision.
How to Use:
- Open Browser
- Observe the canvas.
- Observe the character moving down the canvas.
- Observe the impact of the collision.
Demonstration:
Ojambo.com HTML5 Game Physics Moving Elastic Collision Tutorial
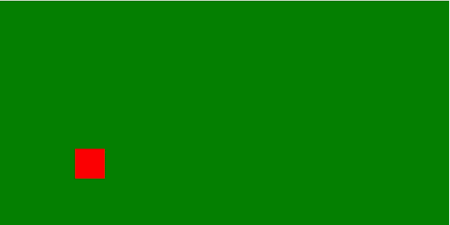
Conclusion:
Game characters have constant acceleration caused by position, velocity and time. The velocity changes by an equal amount every equal time period.
Elastic collisions with constant acceleration maintain their momentum. The exact point of collision impact can be determined by the character’s position and dimensions.
- Recommendations:
- Determine which objects moves after a collision.
- Give the moving objects constant acceleration.
- Constant acceleration requires velocity to change in equal increments over equal time.