Detect Mobile Devices Using PHP
Web designers can utilize the PHP to get information about the browser. HTTP_USER_AGENT is a string that contains contents of the header such as the platform used.
The platform is usually the operating system on desktop browsers. On mobile devices, it might be the device name. The string will be converted to lowercase to make sure that the devices can be detected.
This tutorial uses PHP and Apache as the HTTP Server.
- Tools are required:
- Text editor.
- HTTP Server.
- PHP with HTTP API.
- Browser to view output.
Optional Download and install Geany
Geany is required in order to follow this tutorial. For more information about Geany read Ojambo.com Lightweight Programming Editors.
Mobile-Device-Detect.php file
<?php /* * Mobile-Device-Detect.php * * Copyright 2012 edward <http://ojambo.com> * * This program is free software; you can redistribute it and/or modify * it under the terms of the GNU General Public License as published by * the Free Software Foundation; either version 2 of the License, or * (at your option) any later version. * * This program is distributed in the hope that it will be useful, * but WITHOUT ANY WARRANTY; without even the implied warranty of * MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the * GNU General Public License for more details. * * You should have received a copy of the GNU General Public License * along with this program; if not, write to the Free Software * Foundation, Inc., 51 Franklin Street, Fifth Floor, Boston, * MA 02110-1301, USA. * * */ // Get User Agent $user_agent = strtolower($_SERVER['HTTP_USER_AGENT']); // Values To Check function userAgentContains() { return implode("|", array( 'android', 'blackberry', 'iphone', 'windows' )); } // Check If Mobile Device Matched if (preg_match("/(" . userAgentContains() . ")/i", $user_agent)) { echo "is mobile device"; } else { echo "not mobile device"; } ?>
The PHP tags indicate where the PHP code begins and ends. The user agent is retrieved from the header “HTTP_USER_AGENT” and converted to lowercase. The mobile devices are placed in an array which can be expanded from the example.
The mobile device array is converted into a string divided by a vertical bar or pipe symbol by the function userAgentContains. Finally the user agent string is checked to determine if its content matches any of the predetermined mobile devices. If a match is found, the screen output will be “is mobile device”.
How to Use:
- Open Browser
- Save Mobile-Device-Detect php file in server path.
- Load Mobile-Device-Detect php file.
- Compare output between desktop and mobile browsers.
Demonstration:
Ojambo.com Mobile Device Detect Tutorial

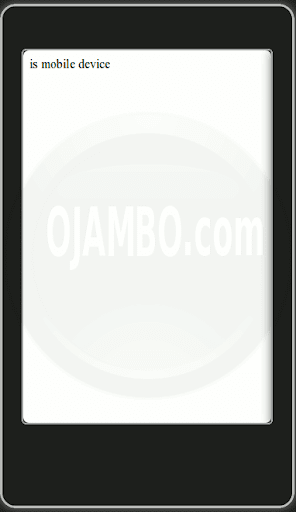
Conclusion:
The HTTP_USER_AGENT user agent string can be used to detect mobile devices. The string can be changed to lowercase or uppercase to eliminate errors in detecting mobile devices. Detect occurs by matching specific devices to content in the user agent string.
PHP runs on the server side. Using PHP to determine mobile devices is cross-browser compatible.
- Recommendations:
- Place your PHP code on the server.
- Mobile detection allows a web designer to present specific stylesheets.
- References:
- Ojambo.com Lightweight Programming Editors.