Build GUI For Desktop Applications Using Node.js And Electron
Node.js is an open-source cross-platform JavaScript runtime environment. Electron is an open source project that embeds Chromium and Node.js to create desktop applications.
Electron is maintained by the OpenJS Foundation which was founded in 2019 from a merger of JS Foundation and Node.js Foundation.
Electron requires a basic understanding of JavaScript, HTML and CSS.
Electron may be used for a future desktop only version of Learning PHP eBook.
This tutorial will create a simple multiplatform GUI application that can be used as a starting template.
For this example, I will assume that Node.js is installed with your preferred package manager. You do not need to know JavaScript or HTML programming, as it is easy to grasp the basic tasks and follow along.
Install Electron Via NPM
npm install -g electron-prebuilt electron --version
Create package.json File
npm init
Name | Description | Answer |
---|---|---|
package name | Application Name | my-electron-app |
version | Application Version | 1.0.0 |
description | Application Description | Hello World! |
entry point | Main File Holding Node.JS handlers | main.js |
test command | Testing command to run before building the application | |
git repository | GIT SCM Repositoru | |
keywords | Keywords relevant to the application for easier searching | |
author | Enter your name | Edward Ojambo |
license | License for the application | MIT |
Is this OK? | Approve displayed output to create package.json | yes |
Manually Add Start Script To package.json File
{ "name": "my-electron-app", "version": "1.0.0", "description": "Hello World!", "main": "main.js", "scripts": { "test": "echo \"Error: no test specified\" && exit 1", "start": "electron ." }, "author": "Edward Ojambo", "license": "MIT" }
Create main.js File
const { app, BrowserWindow } = require('electron/main') const path = require('node:path') function createWindow () { const win = new BrowserWindow({ width: 800, height: 600 }) win.loadFile('index.html') } app.whenReady().then(() => { createWindow() app.on('activate', () => { if (BrowserWindow.getAllWindows().length === 0) { createWindow() } }) }) app.on('window-all-closed', () => { if (process.platform !== 'darwin') { app.quit() } })
Create Static Index.HTML File
<!DOCTYPE html> <html> <head> <meta charset = "UTF-8"> <title>Hello World!</title> </head> <body> <h1>Hello World!</h1> </body> </html
Build And Run
npm start
View
You will see a window open that resembles other desktop applications.
Screenshots:
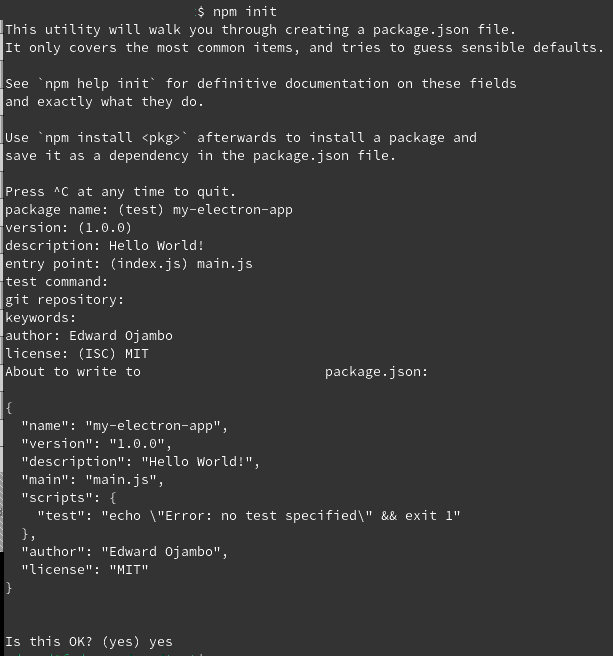
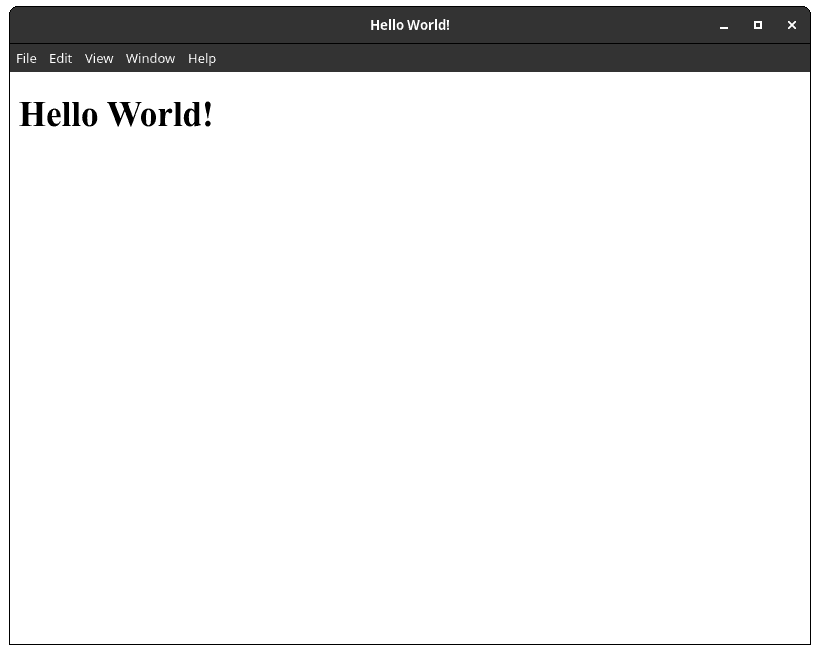
Conclusion:
In summary, Node.js and Electron can be used to create cross platform GUI applications. Node.js with Electron can be used for the GUI portion and the rest of the application can use another platform or programming language.